Oracle mediator is used to route the message from one destination to another but
during integration we do have requirment when we need to call some java code
from mediator and we have provision in mediator to call java code using Java
Callout.
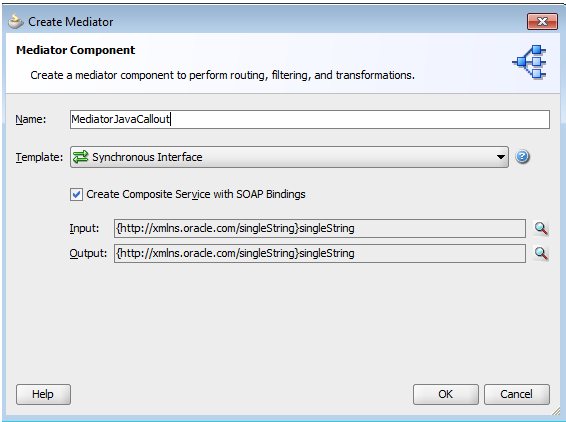
Java Callout is used when we need to have certain valdation or want to
manipulate the message. We need to implement the oracle.tip.mediator.common.api.IjavaCallout interface to use below
defined function for Java Callout.
We have below methods in the oracle.tip.mediator.common.api.IjavaCallout
interface.
·
Initialize : This method is invoked when the callout
implementation class is instantiated for the first time.
·
preRouting : This method is called before Mediator starts
executing the cases. You can customize this method to include validations and
enhancements.
·
preRoutingRule : This method is called before Mediator starts
executing any particular case. You can customize this method to include
case-specific validations and enhancements.
·
preCallbackRouting: This method is called before Mediator
finishes executing callback handling. You can customize this method to perform
callback auditing and custom fault tracking.
·
postRouting : This method is called after Mediator finishes
executing the cases. You can customize this method to perform response auditing
and custom fault tracking.
·
postRoutingRule: This method is called after Mediator starts
executing the cases. You can customize this method to perform response auditing
and custom fault tracking.
·
postCallbackRouting: This method is called after Mediator
finishes executing callback handling. You can customize this method to perform
callback auditing and custom fault tracking.
In this post, I will show you how we can use Java Callout functionality
inside mediator to change request message.
We have prepared one use case, in this use case we call one synchronous
service (SOA composite) from mediator and that synchornous service simple
assign input message to output message. In mediator by using Java Callout we
will modify the input message to synchronous service.
First create a SOA project and add synchronous mediator to it.
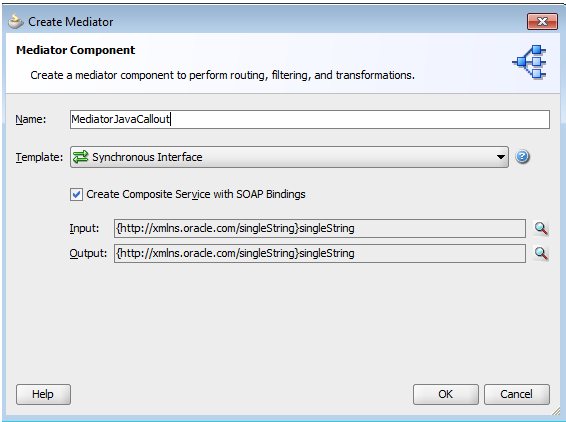
Once you add the mediator, add synchornous service reference in
composite.xml and wire it to mediator.
Now open the mediator .mplan file and add necessary mapping to it.
we are just mapping input message from Mediator to input message of
synchornous service and response message from synchornous service to response
message of mediator.
Now we will add one java class which implements oracle.tip.mediator.common.api.IjavaCallout interface.
To add java class to your project, right click on your project and choose
Java Class and add it. Name your java class.
As we need to implement oracle.tip.mediator.common.api.IjavaCallout
interfce so click on green sign, this will open another pop-up window,
from that window choose IJavaCallout from
oracleà tipà mediatorà commonà api path.
Your class
should look like below.
Once you
create this class, you will see all default methods in your class. You can use required
method as per your requirement.
We will use preRouting method to implement our use
case. In this method we will modify the request message that mediator pass to synchronous
service.
Mykey = “request”
or “reply”: This specify for which transaction
(Request or Response) we are manipulating the message.
As you
noticed in this function we are replacing the input message to “changed text”, so whatever value will
come in singleString element, this java
code replace that with “changed text” and pass it to synchronous service.
Below is the sample preRouting method.
public
boolean preRouting(CalloutMediatorMessage calloutMediatorMessage) throws
MediatorCalloutException {
System.out.println("Pre
routing...");
String sPayload = "null";
String sPayload_changed =
"null";
for (Iterator msgIt =
calloutMediatorMessage.getPayload().entrySet().iterator(); msgIt.hasNext(); ) {
Map.Entry msgEntry = (Map.Entry)msgIt.next();
Object msgKey = msgEntry.getKey();
if
(msgKey.equals("request")) {
Object msgValue =
msgEntry.getValue();
sPayload =
XmlUtils.convertDomNodeToString((Node)msgValue);
try {
XMLDocument xmlpayload =
XmlUtils.getXmlDocument(sPayload);
NodeList node =
xmlpayload.getChildNodes();
Node item = node.item(0);
System.out.println("the value of the request element = " +
item.getTextContent());
sPayload_changed =
sPayload.replaceAll(item.getTextContent(),
"changed text");
XMLDocument
xmlpayload_changed =
XmlUtils.getXmlDocument(sPayload_changed);
String mykey =
"request";
calloutMediatorMessage.addPayload(mykey,
xmlpayload_changed);
System.out.println("Mediator sends request: " +
sPayload_changed);
} catch (Exception e) {
System.out.println(e);
}
}
}
return true;
}
Now we need
to add above generated Java class to mediator. To do that go to mediator .mplan
file and click on callout To sign and choose above generated java class.
Now deploy
your composite and test it.
Testing Results:
awesome post
ReplyDelete